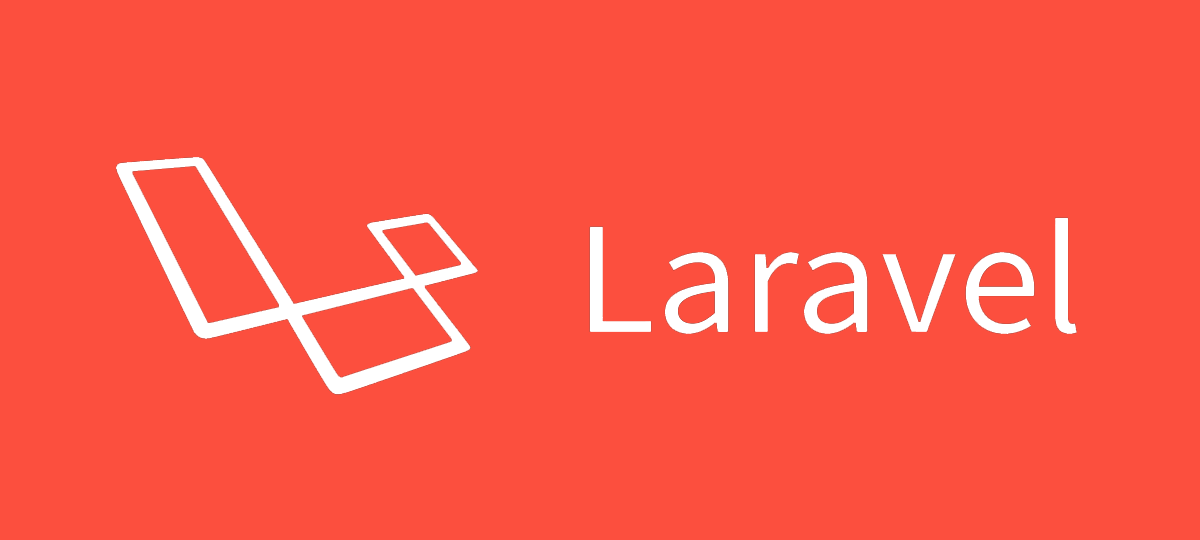
[Laravel] テーブルのプライマリキーにuuidを使う
Laravel5.6で追加されたStr::orderedUuid()
を使って、テーブルのプライマリキーにuuid
を使ってみましょう。
database/migrations/2014_10_12_000000_create_users_table.php
を編集して、users
テーブルのプライマリキーを変更します。
// 変更前
$table->increments('id');
// 変更後
$table->string('id')->primary();
app/User.php
をuuid
を使うように編集します。
オートインクリメントフラグを変更して、Eloquentのcreating
イベントでidにuuid
を代入します。
<?php
namespace App;
use Illuminate\Notifications\Notifiable;
use Illuminate\Foundation\Auth\User as Authenticatable;
class User extends Authenticatable
{
// ...省略
// ここから下を追加
/**
* Indicates if the IDs are auto-incrementing.
*
* @var bool
*/
public $incrementing = false;
/**
* Setup model event hooks
*/
public static function boot()
{
parent::boot();
self::creating(function ($model) {
$model->id = (string) \Illuminate\Support\Str::orderedUuid();
});
}
}
準備ができたのでtinker
からユーザーレコードを作成してみましょう。
$ php artisan tinker
Psy Shell v0.8.17 (PHP 7.1.10 — cli) by Justin Hileman
>>> factory(App\User::class)->create();
Ramsey\Uuid\Exception\UnsatisfiedDependencyException with message 'Cannot call Ramsey\Uuid\Converter\Number\DegradedNumberConverter::toHex without support for large integers, since integer is an unsigned 128-bit integer; Moontoast\Math\BigNumber is required. '
現時点 (v5.6.3) ではStr::orderedUuid()
を利用する為にはパッケージが不足のでインストールします。
$ composer require moontoast/math
それでは気を取り直して。。。
$ php artisan tinker
Psy Shell v0.8.17 (PHP 7.1.10 — cli) by Justin Hileman
>>> factory(App\User::class)->create();
=> App\User {#823
name: "Ms. Winona Christiansen II",
email: "[email protected]",
id: "8a2404c7-585e-47c9-a140-2085b2254da2",
updated_at: "2018-02-17 13:10:36",
created_at: "2018-02-17 13:10:36",
}
>>> factory(App\User::class, 10)->create();
=> Illuminate\Database\Eloquent\Collection {#821
all: [
App\User {#817
name: "Laney Grant",
email: "[email protected]",
id: "8a240832-4a97-4f9b-9121-31164dca93d4",
updated_at: "2018-02-17 13:20:09",
created_at: "2018-02-17 13:20:09",
},
// ...
App\User {#832
name: "Mr. Rasheed Zemlak MD",
email: "[email protected]",
id: "8a240832-543e-4a2b-b72f-1d45bf59efca",
updated_at: "2018-02-17 13:20:09",
created_at: "2018-02-17 13:20:09",
},
],
}
uuid
を使ってユーザーが作成されました!
公式にも記載がありますが、新しく追加されたStr::orderedUuid
を使えば簡単に時系列にそったuuid
が作成できます!
The Str::orderedUuid method generates a "timestamp first" UUID that may be efficiently stored in an indexed database column:
https://laravel.com/docs/5.6/helpers#method-str-ordered-uuid