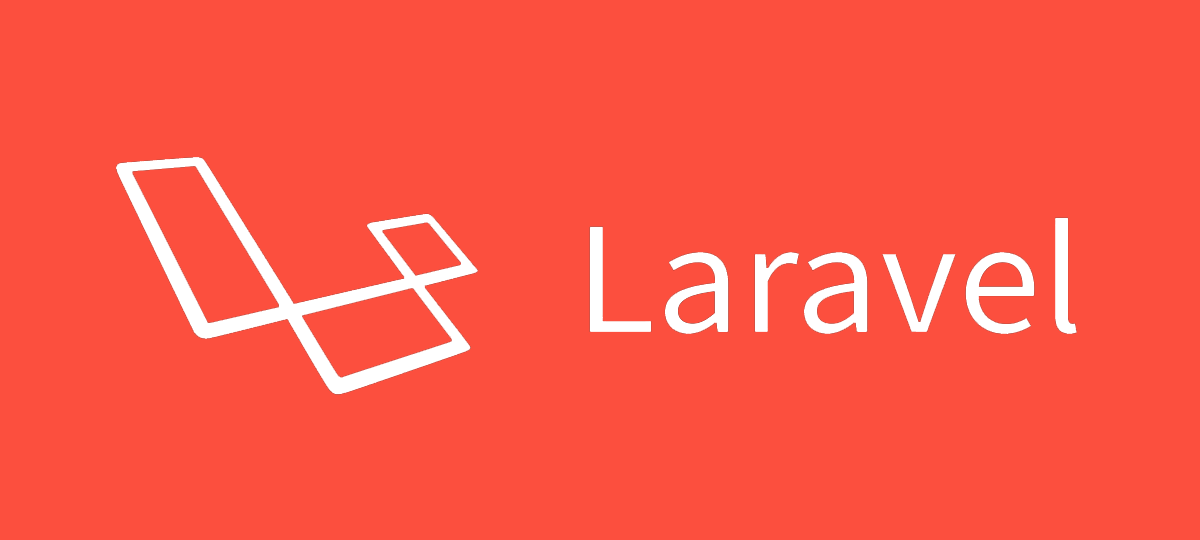
[Laravel] createManyを使用した複数代入
createMany
メソッドの存在を知らなかったのでメモ。
LaravelのEloquentで1対多の関係にあるテーブルに対して複数件のレコードを挿入します。
ユーザーに対して複数のタスクを登録するサンプルです。
ユーザー周りは既存のものをそのまま利用し、タスクモデルとマイグレーションファイルを作成します。
$ php artisan make:model Task --migration
<?php
use Illuminate\Support\Facades\Schema;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Database\Migrations\Migration;
class CreateTasksTable extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('tasks', function (Blueprint $table) {
$table->increments('id');
$table->unsignedInteger('user_id');
$table->string('title');
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::dropIfExists('tasks');
}
}
$ php artisan migrate
Migrating: 2014_10_12_000000_create_users_table
Migrated: 2014_10_12_000000_create_users_table
Migrating: 2017_06_25_000000_create_tasks_table
Migrated: 2017_06_25_000000_create_tasks_table
App/Task.php
<?php
namespace App;
use Illuminate\Database\Eloquent\Model;
class Task extends Model
{
protected $fillable = ['title'];
}
コントローラーを作成してルーティングを変更します。
$ php artisan make:controller CreateManyController
routes/web.php
...
Route::get('/', 'CreateManyController@index');
...
CreateManyController
<?php
namespace App\Http\Controllers;
use App\User;
class CreateManyController extends Controller
{
public function index()
{
$user = factory(\App\User::class)->create();
$user->tasks()->createMany([
['title' => 'First task.'],
['title' => 'Second task.'],
['title' => 'Third task.'],
]);
return view('create_many')->with('users', User::all());
}
}
resources/views/create_many.blade.php
<!doctype html>
<html>
<head>
<title>:CreateMany</title>
</head>
<body>
@foreach($users as $user)
<h2></h2>
<ul>
@foreach($user->tasks as $task)
<li></li>
@endforeach
</ul>
@endforeach
</body>
</html>
/
へアクセスすると、新しいユーザーが作成され、ユーザーに紐づくタスクが追加されます。